OOP Concepts
Abstraction
Abstraction “displays” only the relevant attributes of objects and “hides” the unnecessary details.
For example, when we are driving a car, we are only concerned about driving the car like driving/stopping the car. We are not concerned about how the actual start/stop mechanism process works in the machine.
Encapsulation
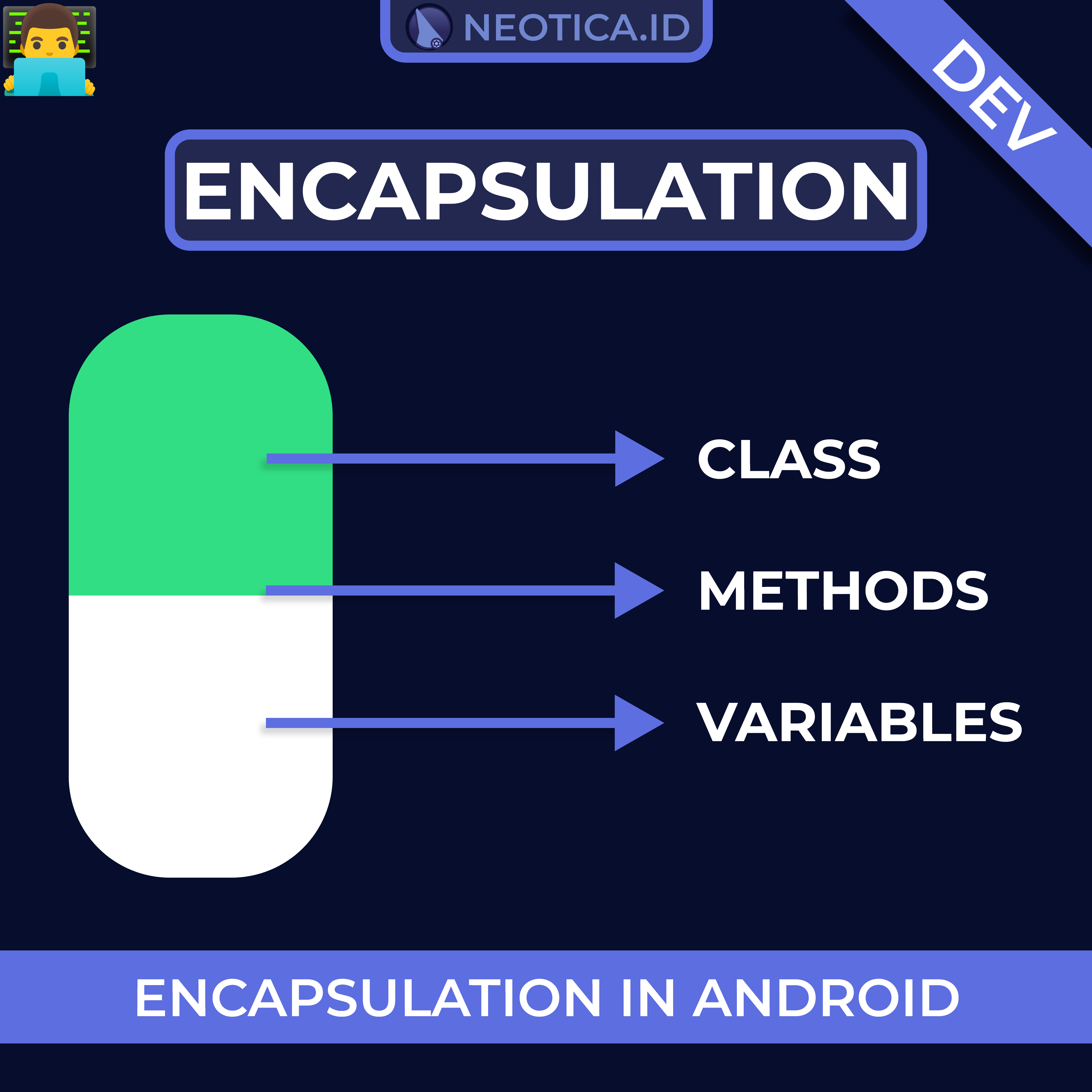
Definition
Encapsulation is a process of hiding the data from the users or in other words we can say it protects the code by preventing access from the outside class., hence it's also called 'Data-Hiding' method.
When implemented, it will provide getter and setter method to be created for object data.
Usage
- Securing data
- Reusable Code
- Easy to test
- Loosely Coupled Code
- Better access control and security
- To provide getter and setter method
Implementing
- Make the instance variables private so that they cannot be accessed directly from outside the class. You can only set and get values of these variables through the methods of the class.
- Have getter and setter methods in the class to set and get the values of the fields.
Example
package.com.neotica
public class Encapsulation {
private String Name;
private String Age;
}
public String getName() {
return Name;
}
public String setName() {
Name = name;
}
public String getAge() {
return Age;
}
public String setAge() {
Age = 12;
}
Using Encapsulation to Strings of variable in java by making it private.
By implementing Getter and Setter method, we will be able to send and receive data externally, without changing the inner codes.
Polymorphism
Usage
- It reduces the complexity of the object.
- Through polymorphism complete implementation can be replaced by using same method signatures.
- It reduces the volume of work in terms of handling various objects.
- Reusable code
Definition
Polymorphism refers to the ability of one thing to take many forms (Poly: many, Morph: forms. It is the property by which same message is send to objects of different classes and the objects behave differently.
Or simply, two objects respond to the same message with different behaviors, and the sender doesn't have to care.
Example
Inheritance
Usage
- It reduces the complexity of the object.
- For Method Overriding (so 'Runtime Polymorphism' can be achieved).
- It reduces the volume of work in terms of handling various objects.
- Reusable code
Definition
Inheritance in Java and Android is a mechanism in which one object acquires all the properties and behaviors of a parent object. It is an important part of OOPs (Object Oriented programming system).
for example, the relationship between father and son. Inheritance in Java is a process of acquiring all the behaviors of a parent object.