Kotlin
Unit in Kotlin corresponds to Void in Java. Like void, Unit is the return type of any function that does not return any meaningful value, and it is optional to mention the Unit as the return type. But unlike void, Unit is a real class (Singleton) with only one instance.
Variables
Var: Mutable Variable
Val: Immutable Variable, like final in Java.
Types are non-null by default in Kotlin. If nullable value is needed, then we can add “?” (Question marks) after the variable types.
val name: String? = null
Kotlin will also recognizes the String value automatically in most cases.
val name = "Kotlin"
var gender = "Non Binary"
Full Code
fun main(){ val name = "Kotlin" var gender = "Non Binary" println("$name $gender") }
Output
Kotlin Non Binary
Expressions
In Kotlin, an expression may be used as a statement or used as an expression depending on the context. As all expressions are valid statements, standalone expressions may be used as single statements or inside code blocks.
If Conditional Expressions
If statements in Kotlin are simple and can be used as a Boolean.
fun main() {
val a = 3
val b = 2
if (a > b) println("a is bigger than b")
else println("a is not bigger than b")
}
Output:
a is bigger than b
When Expressions
When expressions are very similar to Switch statements in Java. The only difference is the When Statements support “Else”(As in If Statements). So that makes the When Statements more flexible to code with.
Example of When expressions when used to replicate an If Conditional:
fun main() {
var age:Int = 10
when(age){
10 -> println("Age is 10")
else -> println("Age is not 10")
}
}
Output:
Age is 10
Functions
Calling functions:
fun getGreeting(): String {
return "Hello Kotlin"
}
fun main() {
println("Hello world")
println(getGreeting())
}
Simplified:
fun getGreeting() = "Hello Kotlin" } fun main() { println("Hello world") println(getGreeting()) }
Output:
Hello World Hello Kotlin
Function Parameter:
fun sayHello(itemToGreet:String){
val msg = "Hello " + itemToGreet
println(msg)
}
fun main(){
sayHello("Kotlin")
}
Simplified:
fun sayHello(itemToGreet:String){ val msg = "Hello $itemToGreet" println(msg) } fun main(){ sayHello("Kotlin") }
More Simplified:
fun sayHello(itemToGreet:String) = println("Hello $itemToGreet") fun main(){ sayHello("Kotlin") }
Output:
Hello Kotlin
Double Parameter:
fun sayHello(greeting:String, itemToGreet:String) = println("$greeting $itemToGreet")
fun main(){
sayHello("Hello", "Kotlin")
}
Output:
Hello Kotlin
The “Hello” is using the first parameter $greeting, and the “Kotlin” is using the second parameter $itemToGreet.
First parameter = $greeting = “Hello”
Second parameter = $itemToGreet = Kotlin
Higher Order Functions
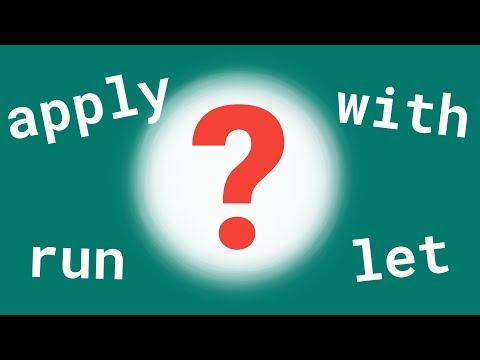
Collections & Iterations
In Kotlin, there’s various types of collections & iterations. Some of which are Arrays, Lists, and Maps.
The basic difference between Array and Lists are: Array is static, while lists are dynamic.
In Collections, Kotlin also differentiate between mutable and immutable collection types.
By defaults, a collection type in Kotlin is immutable.
By immutable it means that you cant add or subtract values from that collections once its initially created.
Arrays
fun main(){
val phone = arrayOf("iPhone", "Pixel", "Samsung")
println(phone.size)
println(phone.get(0))
}
Simplified
fun main(){ val phone = arrayOf("iPhone", "Pixel", "Samsung") println(phone.size) println(phone[0]) }
println(phone.get(0)) = println(phone[0]) Basically, calling string[] in Kotlin is just the same as string.get() in Java.
Lists
fun main(){
val phone = listOf("iPhone", "Pixel", "Samsung")
println(phone.size)
println(phone.get(0))
}
Simplified
fun main(){ val phone = listOf("iPhone", "Pixel", "Samsung") println(phone.size) println(phone[0]) }
Output:
3 iPhone
Show all Arrays
fun main(){
val phone = arrayOf("iPhone", "Pixel", "Samsung")
for (phone in phone){
println(phone)
}
}
Output
iPhone Pixel Samsung
Foreach
Foreach takes in another function and returns Unit. This Foreach method is applicable to Lists as well.
fun main(){
val phone = arrayOf("iPhone", "Pixel", "Samsung")
phone.forEach{
phone -> println(phone)
}
}
Lists Version:
fun main(){ val phone = listOf("iPhone", "Pixel", "Samsung") phone.forEach{ phone -> println(phone) } }
Output:
iPhone Pixel Samsung
It. It is the default name for each element in the array that is passed into this Lambda function in which we are defining.
fun main(){
val phone = arrayOf("iPhone", "Pixel", "Samsung")
phone.forEach{
println(it)
}
}
Output:
iPhone Pixel Samsung
ForEachIndex. ForEachIndex, like its name; is used to find index within Arrays. Use this code to see each Value and which Key are they assigned with, we can use this code.
fun main(){
val phone = arrayOf("iPhone", "Pixel", "Samsung")
phone.forEachIndexed{
index, phone -> println("$phone is at $index")
}
}
Output:
iPhone is at 0 Pixel is at 1 Samsung is at 2
Map
fun main(){
val phone = mapOf(1 to "iPhone", 2 to "Pixel", 3 to "Samsung")
println(phone.size)
println(phone.get(1))
}
Output
3 iPhone
With map you can map and assigns Key to the Value. Like above example, the first Value (“iPhone”) is assigned to Key 1. In default, the Arrays and Lists assigns the first Value to Key 0. But with map, you can reassign every Value into any Keys.
As you can see below, when we requested the Value of Key 0, the output will be Null.
fun main(){
val phone = mapOf(1 to "iPhone", 2 to "Pixel", 3 to "Samsung")
println(phone.size)
println(phone.get(0))
}
Output
3 Null
Map ForEach
Basically ForEachIndex.
fun main(){
val phone = mapOf(1 to "iPhone", 2 to "Pixel", 3 to "Samsung")
phone.forEach{key, value -> println("$key -> $value")}
}
Output
1 -> iPhone 2 -> Pixel 3 -> Samsung
Mutable
Since all collections in Kotlin are immutable by default, to add a mutable collections we have to add functions such as mutableMap.
fun main(){
val phone = mutableMapOf(1 to "iPhone", 2 to "Pixel", 3 to "Samsung")
phone.put(4, "Blackberry")
phone.forEach{key, value -> println("$key -> $value")}
}
Output
1 -> iPhone 2 -> Pixel 3 -> Samsung 4 -> Blackberry
Reference
