Design Pattern
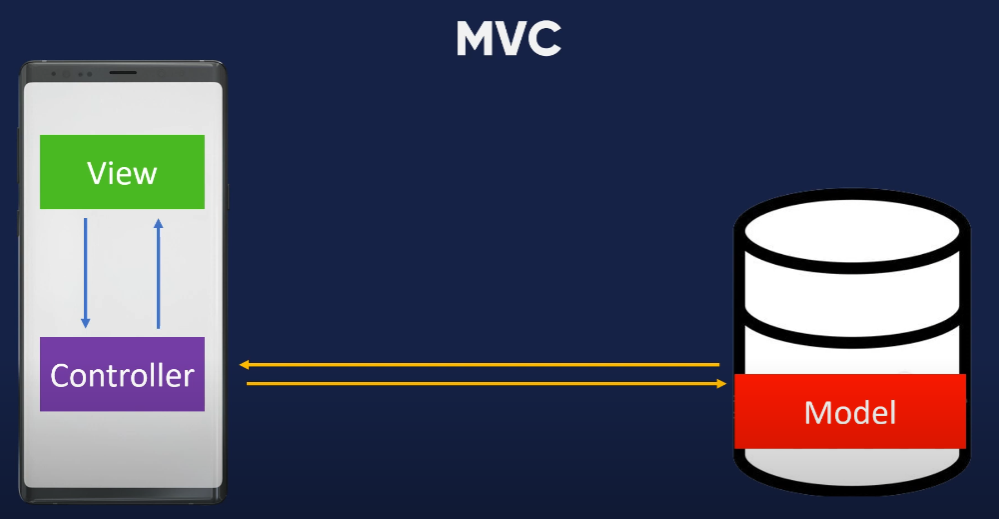
MVC (Model View Controller)
1. You open the refrigerator 2. You get water bottle 3. You pay
Model: Bottle View: You Controller: You
Example: https://github.com/laetuz/MVC-DEMO-ANDROID
1. You request the water 2. Waiter will go to refrigerator 3. You pay and you get the water bottle
Model: Bottle View: You Presenter: The Waiter
Example: https://github.com/laetuz/MVP-DEMO
MVVM (Model View View-Model)
1. You insert the coin 2. You request the water 3. Machine will release the bottle
Model: Bottle View: You View-Model: “BINDING”
How to implement:
- We need to make the connection “Binding” - “ViewModel”
- Implement the ViewModel dependency. Grab it Here.
ViewModel Dependency
dependencies { def lifecycle_version = "2.6.0-alpha01" def arch_version = "2.1.0" // ViewModel implementation "androidx.lifecycle:lifecycle-viewmodel:$lifecycle_version" // LiveData implementation "androidx.lifecycle:lifecycle-livedata:$lifecycle_version"
dependencies { val lifecycle_version = "2.6.0-alpha01" val arch_version = "2.1.0" // ViewModel implementation("androidx.lifecycle:lifecycle-viewmodel:$lifecycle_version") // LiveData implementation("androidx.lifecycle:lifecycle-livedata:$lifecycle_version")
- Create the ViewModel Class.
- Extends the class to the ViewModel.
public class AppViewModel extends ViewModel {
- Connect ViewModel with Model(Or Database in most projects).
private Model getAppFromDatabase(){
return new Model("MVVM DEMO",233,5);
}
- Request LiveData by inputting these codes into ViewModel Class:
MutableLiveData<String> mutableLiveData;
- Connecting ViewModel with MainActivity (View).
public void getAppName(){
String appName = getAppFromDatabase().getAppName();
mutableLiveData.setValue(appName);
}
- Connecting MainActivity with ViewModel and listening to LiveData, in MainActivity
AppViewModel appViewModel;
- Inside the Oncreate, add new ViewModelProvider
appViewModel = new ViewModelProvider(this).get(AppViewModel.class);
- Still inside the OnCreate, Listening and observing the changes to LiveData
appViewModel.mutableLiveData.observe(this, new Observer<String>() {
@Override
public void onChanged(String s) {
textView.setText(s);
}
- Go to AppViewModel.java and we can edit, add data now if we change the code of MutableLiveData to
MutableLiveData<String> mutableLiveData = new MutableLiveData<>();
- Final Result
MainActivity.java
package com.neotica.mvvmdemo; import androidx.appcompat.app.AppCompatActivity; import androidx.lifecycle.Observer; import androidx.lifecycle.ViewModelProvider; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView; public class MainActivity extends AppCompatActivity{ Button button; TextView textView; //6 Connecting MainActivity with ViewModel and listening to LiveData AppViewModel appViewModel; //Linking activity with presenter //Presenter presenter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); button = findViewById(R.id.button); textView = findViewById(R.id.textView); button.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { //Calling the presenter data from Model //No linkage between Activity and Model //MainActivity is handling UI only //All logic occurs at the presenter appViewModel.getAppName(); } }); //6.1 add new ViewModelProvider appViewModel = new ViewModelProvider(this).get(AppViewModel.class); //7 Listening and observing the changes to LiveData appViewModel.mutableLiveData.observe(this, new Observer<String>() { @Override public void onChanged(String s) { textView.setText(s); } }); } //Model: Model.java class //View: Activity with Text & Button //ViewModel: AppViewModel.java //The Presenter and the View is then linked using AppView.java (Interface) //We need to make the connection "Binding" = "ViewModel" }
Model.java
package com.neotica.mvvmdemo; public class Model { //Act as our Database or Cloud Storage //This is the model in MVP String appName; int appDownloads; int appRating; // Constructor public Model(String appName, int appDownloads, int appRating) { this.appName = appName; this.appDownloads = appDownloads; this.appRating = appRating; } // Getter and Setter public String getAppName() { return appName; } public void setAppName(String appName) { this.appName = appName; } public int getAppDownloads() { return appDownloads; } public void setAppDownloads(int appDownloads) { this.appDownloads = appDownloads; } public int getAppRating() { return appRating; } public void setAppRating(int appRating) { this.appRating = appRating; } }
AppViewModel.java
package com.neotica.mvvmdemo; import androidx.lifecycle.MutableLiveData; import androidx.lifecycle.ViewModel; public class AppViewModel extends ViewModel { //4. LiveData MutableLiveData<String> mutableLiveData = new MutableLiveData<>(); //3. Connect ViewModel with Model(Or Database in most projects). private Model getAppFromDatabase(){ return new Model("MVVM DEMO",233,5); } //5. Connecting ViewModel with MainActivity public void getAppName(){ String appName = getAppFromDatabase().getAppName(); mutableLiveData.setValue(appName); } }
Source Code: https://github.com/laetuz/MVVM-DEMO-ANDROID-JAVA
Reference:
MVVM on Kotlin:
MVVM on Android Crash Course - Kotlin & Android Architecture Components - Reso Coder
Model - View - ViewModel is an architectural pattern which will empower you to write manageable, maintainable, cleaner and testable code. MVVM is also supported and encouraged by Google itself. There are many first-party libraries like lifecycle-aware components, LiveData, ViewModel and many more. In the previous post, you learned the theory behind MVVM.

